Secure Data Handling with Rails Encrypted Attributes
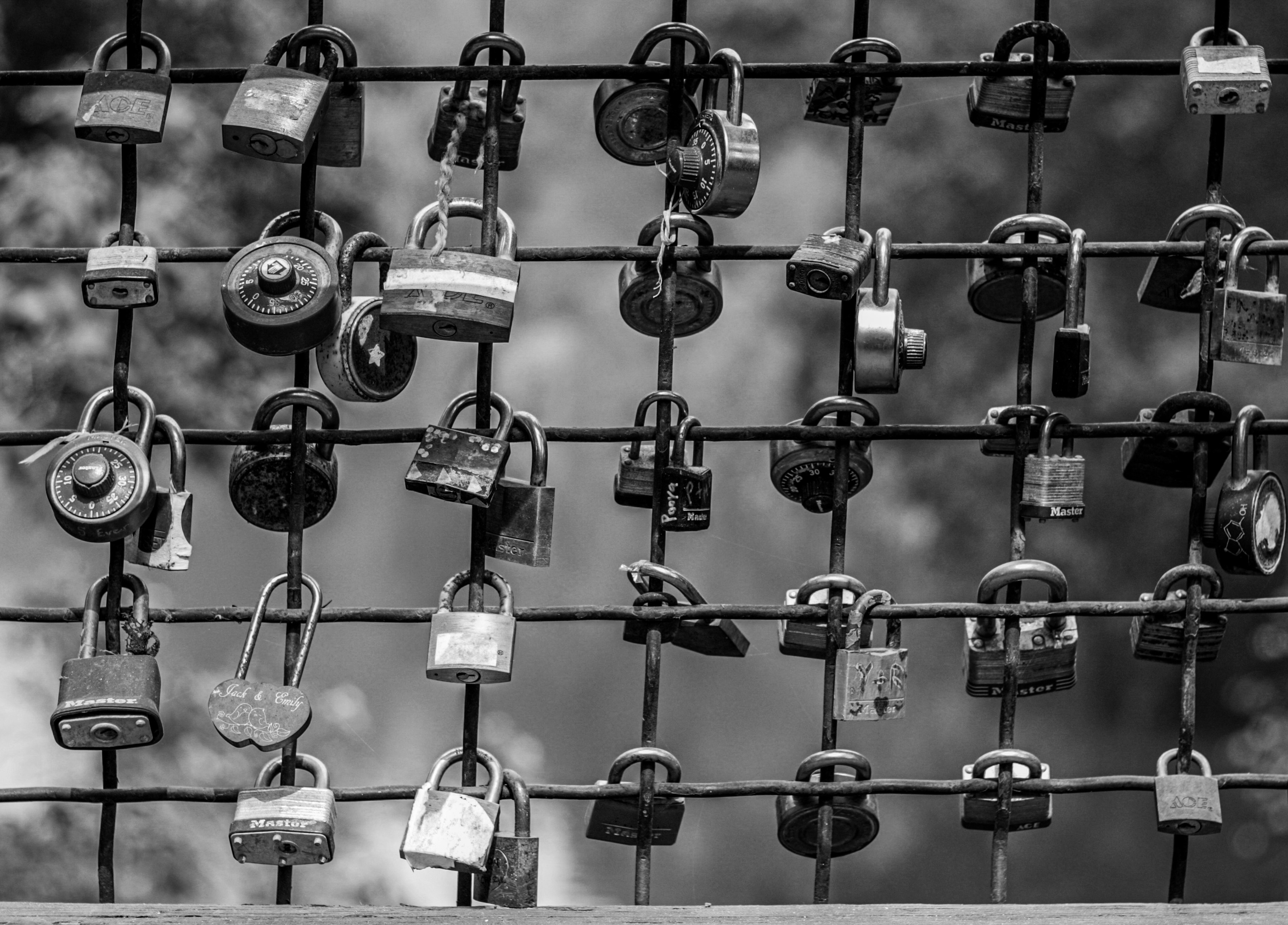
While working on a Rails project, I needed to store customer data securely. I wanted a simple, built-in solution without adding unnecessary complexity.
That’s when I discovered Rails’ built-in encryption for Active Record attributes. With just a few lines of code, I could encrypt and decrypt data seamlessly. It was easy to implement, required no extra dependencies, and worked effortlessly. After seeing how effective it was, I knew this was something worth sharing.
Why Use Rails Encrypted Attributes?
Stronger Security: Even if someone accesses your database, encrypted data remains unreadable.
Easy to Implement: Rails handles encryption and decryption automatically.
Built-in Support: No extra gems or external services needed; introduced in Rails 7.0.
Basic Implementation
To implement encryption in a Customer
model, you can use:
class Customer < ApplicationRecord
encrypts :payment_details
encrypts :customer_email
end
Rails automatically encrypts data when saved and decrypts it when retrieved.
How It Works
Encryption Keys: Rails stores a master key securely in
config/credentials.yml.enc
.Transparent Encryption: Data is encrypted before saving.
Automatic Decryption: Data is decrypted seamlessly when accessed.
Stored Data Example
Here’s how encrypted data appears in the database versus its original form:
Before Encryption (Plain Data):
id: 1
customer_email: customer@example.com
payment_details: 4111-1111-1111-1111
After Encryption (Stored in Database):
id: 1
customer_email: Cq2fM+G1Lq6YbV...==
payment_details: xR9KmA7ZdXw==...
As you can see, the encrypted attributes are transformed into unreadable strings, ensuring that sensitive data remains protected.
Further Reading
For more details, including how to migrate existing unencrypted data, check the official Rails documentation: Ruby on Rails Guides - Active Record Encryption.
Credits
Photo by Parsoa Khorsand on Unsplash.